All tutorials
Using the Web Map Component
We are pleased to announce the public release of the Web Map Component.
Kindly submit any feedback to our Support team at support@what3words.com.
Introduction
The easiest way to add what3words to your technology is to use one of our components. We have several components available for JavaScript, iOS, and Android which require minimal development to integrate. They can also be used in Angular, React, or Vue projects as framework-native components. Find out how else you can utilise the what3words API using JavaScript by taking a look at the functions in the what3words JavaScript API Wrapper. The web map component declares a custom DOM element that allows for a quick and simple way of adding the what3words grid on top of Google Maps, displaying the what3words address and marker on the map for a selected three word address.
You can optionally integrate the AutoSuggest Component with the map too and it will enable searching of 3 word addresses or traditional addresses and traverses the map to the selected three word address. The component by default uses the what3words API or can be configured to use a privately hosted instance of the what3words API. This tutorial steps through how our JavaScript Map Component can be added to a website either for static pages via our CDN or using NPM as part of your project dependencies.
Follow the steps below to select your chosen technology to find out how you can add the what3words map component to your project.
Follow the steps below to select your chosen technology to find out how you can add the what3words map component to your project.
Quickstart
The Web Map Component requires just a few lines of code to be added to a site to create a map with what3words functionality. Replace YOUR-API-KEY
with your what3words API key and the YOUR-GOOGLE-API-KEY
with your Google Maps API key.
<head> <script type="module" defer src="https://cdn.what3words.com/javascript-components@4.8.0/dist/what3words/what3words.esm.js"></script> <script nomodule defer src="https://cdn.what3words.com/javascript-components@4.8.0/dist/what3words/what3words.js"></script> <style> html, body { margin: 0px; height: 100%; } #map-container { width: 100vw; height: 100vh; } #search-container { margin: 10px 0 0 10px; } #search-input { width: 300px; } #current-location-container { margin: 0 10px 10px 0; } </style> </head> <body> <what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-MAP-API-KEY" disable_default_ui fullscreen_control map_type_control zoom_control current_location_control_position="9" fullscreen_control_position="3" search_control_position="2" words="filled.count.soap" > <div slot="map" id="map-container"></div> <div slot="search-control" id="search-container"> <what3words-autosuggest> <input id="search-input" type="text" placeholder="Find your address" autocomplete="off" /> </what3words-autosuggest> </div> <div slot="current-location-control" id="current-location-container"> <button>Current Location</button> </div> </what3words-map> </body>
Step by Step
Get Google API key
You also will need to get a Google API key for your chosen map provider (only Google is currently supported).
Note:
In console.cloud.google.com you should select: API and services
> Enabled APIs and services
Search for places API
and then select and enable it.
Add Map Component
The component can be added to static HTML pages by including the what3words.js
script on each page of your site that you would like to use the component on. A notable limitation imposed by Google Maps restricts the number of maps that can be rendered on any single page (details are provided in the Troubleshooting
section).
You will need to replace <YOUR-API-KEY>
with your what3words API key. This will attach the what3words API to the window
accessible via window.what3words
. This parameter is only required if you need the latter functionality. Alternatively, if you are using a package manager (e.g. npm
) within your site you can consume one of our packages for your framework.
<script type="module" src="https://cdn.what3words.com/javascript-components@4.8.0/dist/what3words/what3words.esm.js?"></script> <script nomodule src="https://cdn.what3words.com/javascript-components@4.8.0/dist/what3words/what3words.js?key=<YOUR-API-KEY>" defer></script>
Add Current Location Functionality (Optional)
To enable your customers to choose their current location and discover their three word address, you will need to add a current-location-control
slot container with a child button as a sibling to the search-control
slot. This can be styled using CSS with its position on the map canvas controlled by the current_location_control_position
What3Words Map Component property. A simple implementation is shown below:
... <div slot="search-control" id="search-container"> <what3words-autosuggest> <input id="search-input" type="text" placeholder="Find your address" autocomplete="off"> </what3words-autosuggest> </div> <div slot="current-location-control" id="current-location-container"> <button>Current Location</button> </div> ...
Insert Map Component
Then we will need to wrap the map container and the search box within the what3words Map Component as shown here to display the what3words grid and marker on Google Maps. Notice you will need to add your what3words API key to the api_key
parameter and your Google Maps API key to map_api_key
parameter.
Note: You will also need to add an import
line to our package if you are using npm.
<body> <what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-API-KEY" > </what3words-map> </body>
Define a map container
Add HTML and CSS to create a page with a #map
element. The #map
is the element that displays the map and its CSS resets any browser settings, so it can consume the full width and height of the browser. Note that we must specifically declare those percentages for <body> and <html> as well.
For the map to display on a web page, we must reserve a spot for it. Commonly, we do this by creating a named <div> element and obtaining a reference to this element in the browser’s document object model (DOM).
<body> <what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-API-KEY" > <div slot="map" id="map"></div> </what3words-map> </body>
Insert Search field
You could insert a seach box by simply adding a search-control slot
within the Map Component. In the search-control slot
you can specify your customised search box.
<body> <what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-API-KEY" > <div slot="map" id="map"></div> <div slot="search-control"> // Enter here your customised Search box </div> </what3words-map> </body>
Use AutoSuggest Component in the Search field (Optional)
You could also provide a type-ahead search box that includes the what3words Web AutoSuggest Component and the common address from Google Places, you will need to add the following code on your body below the div
element that is used as a map container.
Note: This is completly optional. You will also need to add an import
line to our AutoSuggest Component package if you are using npm
.
<body> <what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-API-KEY" > <div slot="map" id="map"></div> <div slot="search-control"> <what3words-autosuggest> <input type="text" placeholder="e.g. ///filled.count.soap" /> </what3words-autosuggest> </div> </what3words-map> </body>
Customise the Map Component
The Map Component can be customised using common Google Maps options and other what3words properties. Here is shown an example of how to add these options and properties together.
<what3words-map id="w3w-map" api_key="YOUR-API-KEY" map_api_key="YOUR-GOOGLE-API-KEY" zoom="8" selected_zoom="20" lng="-0.114637" lat="51.454843" search_control_position="2" map_type_control zoom_control fullscreen_control fullscreen_control_position="3" current_location_control_position="9" disable_default_ui map_type_id="satellite" words="filled.count.soap" > ... </what3words-map>
Configuring for use with API server
If you run our Enterprise Suite API Server yourself, you may specify the URL to your own server by adding the base_url
parameter to the Map Component.
<what3words-map ... base_url="https://example.com/v3" > ... </what3words-map>
Styling Map Component
By default, the Web Map Component will load with the what3words predefined map marker icon. You can choose your own icon by adding their URL path to the marker_icon
attribute.
<what3words-map ... marker_icon="./assets/redmarker.png" > ... </what3words-map>
Select Map Provider
Our Map Component could support different map service providers, such as Google Maps, Mapbox, Apple Maps, and many more, by adding the property provider
to the Map Component. Currently, the only map service provider accepted is Google Maps provider = "google"
but in the future will be extended to support mapbox
(for MapBox), apple
(for Apple Maps) and other map providers.
<what3words-map ... provider="google" > ... </what3words-map>
Add Strict mode
You can now add strict=false
or just strict
if you want it to be true (which is the default) to the AutoSuggest Component which allows for non-3wa-like strings to be accepted and the component will not remove the value. It will emit the value_invalid
event as it normally does, but the value is not removed and can be used to trigger another integration, such as Google Places API, which the map component does. This way you can now use the autosuggest component with an existing field rather than having to have a dedicated field on your form.
Customise Suggestions (Optional)
If you are using our AutoSuggest Component in your Search box, with the new alpha build you can also push your own suggestions to the AutoSuggest Component using the option
attribute or programmatically updating this with JavaScript as is shown in the example below. This is currently available through the React and Vue wrappers.
import { What3wordsAutosuggest } from "@what3words/react-components"; import { createRef, useEffect } from "react"; import "./styles.css"; export default function App() { const ref = createRef(); useEffect(() => { ref.current.addEventListener("value_invalid", () => { const options = [ { value: "Street A", id: "street-a", description: "Some village", distance: { value: 10, units: "km" } }, { value: "Street B", id: "street-b", description: "Some town", distance: { value: 30, units: "km" } }, { value: "Street C", id: "street-c", description: "Some city", distance: { value: 90, units: "metres" } } ]; ref.current.options = options; }); return () => ref.current.removeEventListener("value_invalid", () => { console.log("here"); }); }, [ref]); return ( <What3wordsAutosuggest ref={ref} api_key="YOUR-API-KEY"> <input type="text" /> </What3wordsAutosuggest> ); }
Attributes
Component script parameters
Parameter | Type | Description |
---|---|---|
key | String | An API key is required for the component to function correctly. Example: key=Your-API-Key |
baseUrl | String | The component can be used with an Enterprise Suite API Server instance by using baseUrl. Example: baseUrl=https://example.com/v3 |
callback | String | Allows for a callback to be fired once the component has loaded. This should be the function name to be fired. Example: callback=initMap |
headers | String | Enterprise API Server only - Allows for custom headers to be passed through in requests. |
Component Attributes
Parameter | Type | Description |
---|---|---|
api_key | String | Your what3words API Key can be specified as an attribute to the component. Example: api_key="Your-API-Key" |
api_version | String | You can specify the what3words API version as an attribute to the component. Example: api_version=”v1” |
base_url | String | The component can be used with an Enterprise Suite API Server instance by using base_url. Example: base_url =https://example.com/v3 |
current_location | Boolean | Enables/disables the current location. |
current_location_control_position | Number | Position id. Used to specify the position of the current location control on the map. |
disable_default_ui | Boolean | Enables/disables all default UI buttons. May be overridden individually. Does not disable the keyboard controls or the gesture controls. |
fullscreen_control | Boolean | The enabled/disabled state of the Fullscreen control. |
fullscreen_control_position | Number | Position Id. Used to specify the position of the control on the map. The default position is RIGHT_TOP |
headers | String | Enterprise API Server only - Allows for custom headers to be passed through in requests. |
language | String | See https://developers.google.com/maps/faq#languagesupport Allows you to add a preferred suggestion fallback language for google maps. Example: language="EN" |
lat | Number | It specifies the latitude of an initial position on the map. Example: lat=-34.397. |
libraries | ("drawing" | "geometry" | "localContext" | "places" | "visualization") | It loads additional libraries by specifying a libraries parameter in the request, and supplying the name of the library or libraries. You can specify multiple libraries as a comma-separated list. See the full list here: https://developers.google.com/maps/documentation/javascript/libraries. |
lng | Number | It specifies the longitude of an initial position on the map. Example: lng=150.644 |
map_api_key | String | A Google Maps API key is required for the component to function correctly. |
map_type_control | Boolean | The initial enabled/disabled state of the Map type control. |
map_type_control_position | Number | Position id. Used to specify the position of the map type control on the map. Default TOP_RIGHT. |
map_type_id | "hybrid" | "roadmap" | "satellite" | "terrain" | IDs of map types to show in the control. |
marker_icon | String | You can use the default marker icon or use your own one [MARKER_SRC]. Example: marker_icon="logo_sample.jpg". |
provider | String | Map Service provider. Currently it accepts only "google". In the future will be extended to support "mapbox", "apple" and other map service providers. |
region | String | A region code of the user's region. The region code accepts a ccTLD ("top-level domain") two-character value. Most ccTLD codes are identical to ISO 3166-1 codes, with some notable exceptions. For example, the United Kingdom's ccTLD is "uk" (.co.uk) while its ISO 3166-1 code is "gb" (technically for the entity of "The United Kingdom of Great Britain and Northern Ireland"). |
rotate_control | Boolean | The enabled/disabled state of the Rotate control. |
rotate_control_position | Number | Position id. Used to specify the position of the rotate control on the map. The default position is TOP_LEFT. |
scale_control | Boolean | The initial enabled/disabled state of the Scale control. |
search_control_position | Number | Position id. Used to specify the position of the search control on the map. The default position is TOP_LEFT. |
selected_zoom | Number | The initial what3words Grid zoom level. |
street_view_control | Boolean | The initial enabled/disabled state of the Street View Pegman control. This control is part of the default UI, and should be set to false when displaying a map type on which the Street View road overlay should not appear (e.g. a non-Earth map type). |
street_view_control_position | Number | Position id. Used to specify the position of the street view control on the map. The default position is embedded within the navigation (zoom and pan) controls. If this position is empty or the same as that specified in the zoom_control_position, the Street View control will be displayed as part of the navigation controls. Otherwise, it will be displayed separately. |
tilt | Number | For vector maps, sets the angle of incidence of the map. The allowed values are restricted depending on the zoom level of the map. For raster maps, controls the automatic switching behavior for the angle of incidence of the map. The only allowed values are 0 and 45. |
version | String | Google Maps JavaScript API version loaded by the browser. See https://developers.google.com/maps/documentation/javascript/versions. |
watch_location | Boolean | It watches your location and the event listener will be triggered every time their location changes. |
words | String | Inital what3words address to be searched and displayed on the map. |
zoom | Number | The initial Map zoom level. Valid zoom values are numbers from zero up to the supported maximum zoom level. Larger zoom values correspond to a higher resolution. |
zoom_control | Boolean | The enabled/disabled state of the Zoom control. |
zoom_control_position | Number | Position id. Used to specify the position of the zoom control on the map. The default position is TOP_LEFT. More on the ControlPosition constants on Google Maps:https://developers.google.com/maps/documentation/javascript/reference/control#ControlPosition. |
Events
Event | Description |
---|---|
coordinates_changed | Fires when coordinates change when a new valid 3 word address is selected. Returns the coordinates of the selected square on the map. |
selected_square | Fires when a square on the what3words grid has been selected. Returns all details of that selected square. |
Listening for events
Using an event listener, it is possible to fire other functionality in a site when something changes in the Map Component. For example, it is possible to listen for when a square on the grid map has been selected by the users or to listen for when new coordinates have been returned and pan/zoom a map to the location.
<script> const w3wMap = document.getElementById("w3w-map"); w3wMap.addEventListener( "selected_square", console.log("Selected what3words square") ); </script>
Methods
Method Name | Results |
---|---|
clearGrid | clearGrid(); Parameters: None. Return Value: None. Reset the polilynes of the grid on the map. |
getBounds | getBounds(); Parameters: None. Return Value: LatLngBounds|undefined. Returns the lat/lng bounds of the current viewport [south: number; west: number; north: number; east: number;]. If the map is not yet initialized or center and zoom have not been set then the result is undefined. |
getGrid | getGrid(); Parameters: None. Return Value: google.maps.Polyline[]. Returns the polylines of the grid on the map. |
getLat | getLat(); Parameters: None. Return Value: number|undefined. Returns the latitude of an initial position on the map. If the latitude has not been set then the result is undefined. |
getLng | getLng(); Parameters: None. Return Value: number|undefined. Returns the longitude of an initial position on the map. If the longitude has not been set then the result is undefined. |
getMapTypeId | getMapTypeId(); Parameters: None. Return Value: MapTypeId|string|undefined. Returns the MapTypeId of the map. |
getZoom | getZoom(); Parameters: None. Return Value: number|undefined. Returns the zoom of the map. If the zoom has not been set then the result is undefined. |
panTo | panTo(coordinates); Parameters: coordinates: LatLng|LatLngLiteral. The new center latitude/longitude of the map. Return Value: None. Changes the center of the map to the given coordinates. If the change is less than both the width and height of the map, the transition will be smoothly animated. |
setApiKey | setApiKey(key); Parameters: key: string. Return Value: None. |
setApiVersion | setApiVersion(api_version); Parameters: api_version: ApiVersion. Return Value: None. |
setBaseUrl | setBaseUrl(host); Parameters: host: string. Return Value: None |
setGrid | setGrid(grid); Parameters: grid: any[]. Return Value: None. |
setHeaders | setHeaders(value); Parameters: value: string. Enterprise API Server only - Allows for custom headers to be passed through in requests. Return Value: None. |
setLat | setLat(lat); Parameters: lat: number. It specifies the latitude of an initial position on the map. Return Value: None. |
setLng | setLng(lng); Parameters: lng: number. It specifies the longitude of an initial position on the map. Return Value: None. |
setMapTypeId | setMapTypeId(mapTypeId); Parameters: mapTypeId: MapTypeId|string. Return Value: None. |
setOptions | setOptions(options); Parameters: options: MapOptions optional. Return Value: None. |
setWords | setWords(words); Parameters: words: string. Initial what3words address to be searched and displayed on the map. Return Value: None. Sets the initial what3words address on the map. |
setZoom | setZoom(zoom); Parameters: zoom: number. Larger zoom values correspond to a higher resolution. Return Value: None. Sets the zoom of the map. |
Full Example in HTML
The example below takes the concepts described earlier and demonstrates how our JavaScript Map Component can be added to a website as an HTML static page via our CDN.
Full Example in React
The example below takes the concepts described earlier and demonstrates how our JavaScript Map Component can be added to a website using NPM as part of your project dependencies and React as a framework.
Full Example in Vue
The example below takes the concepts described earlier and demonstrates how our JavaScript Map Component can be added to a website using NPM as part of your project dependencies and Vue as a framework.
Full Example in Angular
The example below takes the concepts described earlier and demonstrates how our JavaScript Map Component can be added to a website using NPM as part of your project dependencies and Angular as a framework.
Full Example in Angular with Routers
The example below takes the concepts described earlier and demonstrates how our JavaScript Map Component can be added to a website using NPM as part of your project dependencies and Angular as a framework.
If you are looking to build Single Page Applications with multiple views and allow navigation between these views you should use this code snippet to add the Angular Router.
Troubleshooting
“For Development Purposes Only” on Google Maps
Issue
If you see a “For development purposes only” text over your Google Maps and it’s not loading correctly, there’s an issue with your Google Maps API key. This typically occurs because Google Cloud Billing is not enabled, or a Google Maps API key has not been generated for your organization.
Solution
1. Enable Google Cloud Billing:
- Go to your Google Cloud Billing settings and ensure billing is enabled.
- Select your current project at the top left if necessary.
Google requires billing to be enabled if your free trial has ended or if you’ve exceeded the free monthly tier.
2. Generate a Google Maps API Key:
- Follow the instructions in the guide: How to add a Google Maps API key to your store locator.
For further assistance, contact Google Cloud support.
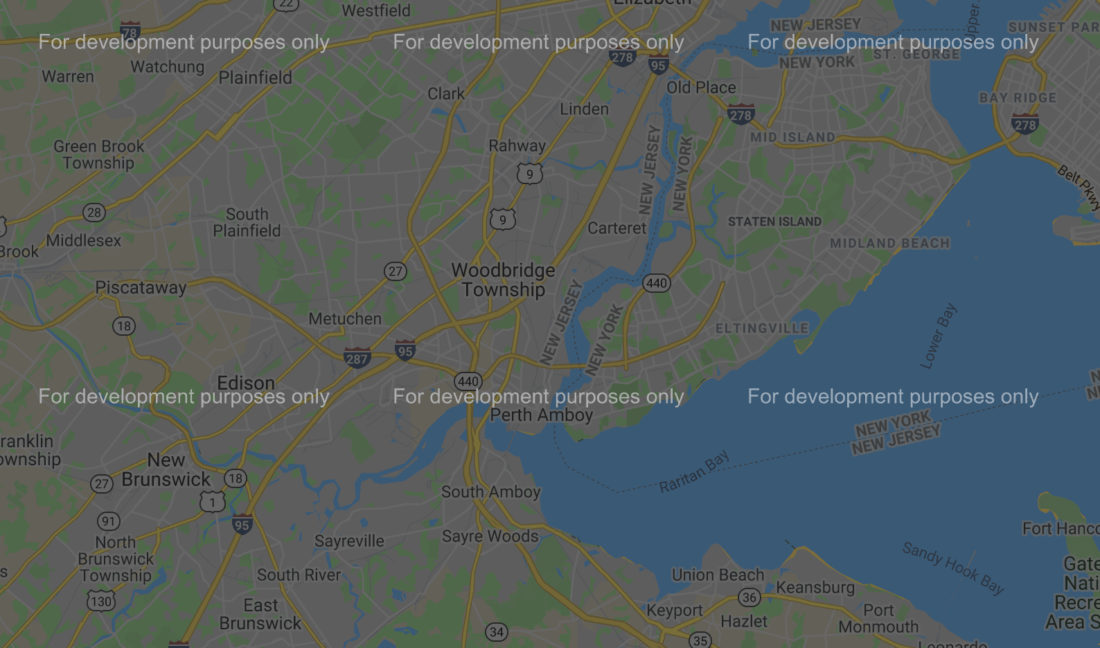
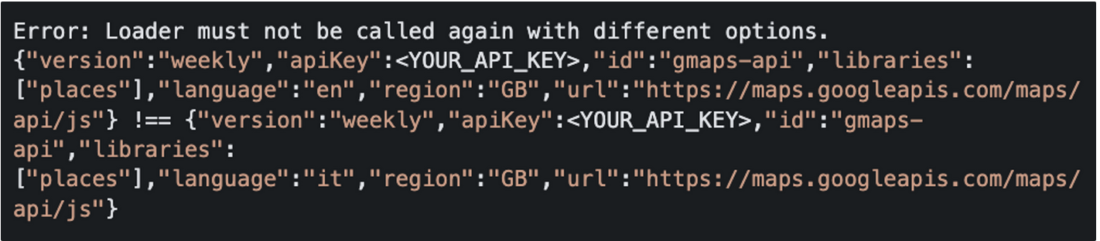
Vite Compatibility Issue
Our current components do not yet work with Vite due to a known issue with Stencil. A potential fix is expected in a future version upgrade, which we are currently working on. It may take some time before this fix is available and tested. For now, we advise against using Vite. For more details, refer to the GitHub issue.